Add two numbers or two integers in java program. This java program perform in different ways and different examples to better understand to the users. These three different ways are without user input, user input at the command line, input at run time. These ways are divided according to the assign the value of two number or two integer or two variable.
Enter a second no:10
Addition of two number are:30
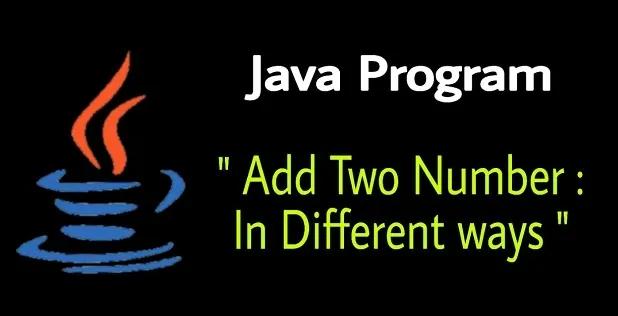
Addition of two number without user input:
Already assign a value of two number at the time of programming. User compile and run the program then show the sum of two number in the output screen.Describe the following program:
Create a class , write main method ,under the main method declare variable with data type, assign value on variable, add two num1 and num2 and print the result .User first open command prompt compile and after run this program.class Addition
{
public static void main(String args[])
{
int num1=10,num2=15,sum;
sum=num1+num2;
System.out.print("Sum of two numbers="+sum);
}
}
Output:
Sum of two numbers=25Addition of two number user input at the command line:
Enter the value of two number at the command line then show the sum of these
two number in the output screen.
class Addition { public static void main(String args[]) { int num1,num2; num1 = Integer.parseInt(args[0]); num2 = Integer.parseInt(args[1]); System.out.println("Sum of two numbers="+(num1+num2)); }}
Output:
Sum of two numbers=50Addition of two number with user input at run time:
Enter the value of two number at the run time then show the sum of these two number in the output screen.
import java.util.Scanner;
class Addition
{
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
int num1,num2,sum;
System.out.print("Enter a first no:");
num1 = sc.nextInt();
System.out.print("Enter a second no:");
num2 = sc.nextInt();
sum=num1+num2;
System.out.print("Addition of two number are :"+sum);
}
}
Output:
Enter a first no:20Enter a second no:10
Addition of two number are:30
1 Comments
So goods
ReplyDelete