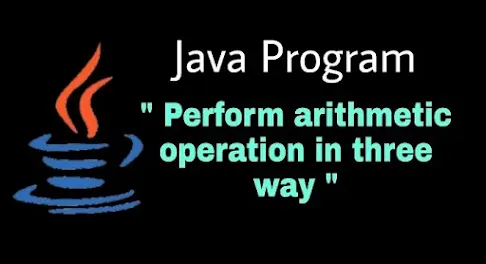
Three ways to perform arithmetic operation:
- Without user input
- With user input at runtime
- With user input at command line
Arithmetic operation without user input:
It means at the time of declaration of variable, value is assign on a and b variable. ‘a’ is an one variable and ‘b’ is another variable. ‘arithmetic’ is a class name.class arithmetic
{
public static void main (String args [] )
{
int a=15, b=12;
System.out.println("Addition of a and b = "+(a+b));
System.out.println("Subtraction of a and b = "+(a-b));
System.out.println("Multiplication of and b = "+(m*n));
System.out.println("Division of a and b = "+(m/n));
System.out.println("Remainder of a and b= "+(m%n));
}
}
Output:
Addition of a and b = 27Subtraction of a and b = 3
Multiplication of and b = 180
Division of a and b = 1
Remainder of a and b= 3
Arithmetic operation with user input at run time:
It means at the execution time the value is assign on a and b variable.import java.util.*;
class arithmetic
{
public static void main (String args [])
{
int a,b;
Scanner sc= new Scanner(System.in);
System.out.print("Enter two no a and b is :");
a=sc.nextInt();
b=sc.nextInt();
System.out.println("Addition of a and b ="+(a+b));
System.out.println("Subtraction of a and b ="+(a-b));
System.out.println("Multiplication of and b ="+(a*b));
System.out.println("Division of a and b ="+(a/b));
System.out.println("Remainder of a and b="+(a%b));
}
}
Output:
Enter two no a and b is : 20 15Addition of a and b = 35
Subtraction of a and b = 5
Multiplication of and b = 300
Division of a and b = 1
Remainder of a and b= 5
Arithmetic operation with user input at command line :
It means the value is assign on a and b variable at the command line.class arithmetic
{
public static void main (String args [])
{
int a,b;a=Integer.parseInt(args[0]);
b=Integer.parseInt(args[1]);
System.out.println("Addition of a and b ="+(a+b))
System.out.println("Subtraction of a and b ="+(a-b));
System.out.println("Multiplication of and b ="+(a*b));
System.out.println("Division of a and b ="+(a/b));
System.out.println("Remainder of a and b="+(a%b));
}
}
Output:
c:\>java arithmetic 30 25Addition of a and b = 55
Subtraction of a and b = 5
Multiplication of and b = 750
Division of a and b = 1
Remainder of a and b= 5
0 Comments