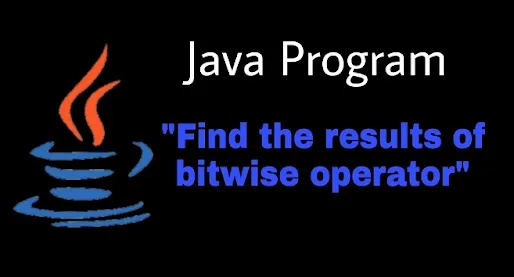
Three ways to perform bitwise operators:
- Without user input
- With user input at runtime
- With user input at command line
Find the result of bitwise operators without user input:
It means at the time of declaration of variable , value is assign on a and b variable. ‘a’ is an one variable and ‘b’ is another variable. ‘math’ is a class name.Class Math
{
public static void main(String args[])
{
int a=15, b=12;
System.out.println("Result of logical AND = "+(m&n));
System.out.println("Result of logical OR = "+(m|n));
System.out.println("Result of logical NOT = "+(~m));
System.out.println("Result of shift right = "+(m>>1));
System.out.println("Result of shift left = "+(m<<1));
System.out.println("a equal to b is : "+(m==n));
}
}
Output:
Result of logical AND = 0Result of logical OR = 15
Result of logical NOT = -6
Result of shift right = 2
Result of shift left = 10
a equal to b is : false
Find the result of bitwise operators with user input at run time:
It means at the execution time the value is assign on a and b variable.import java.util.*;
class math
{
public static void main(String args[])
{
int a,b;
Scanner sc= new Scanner(System.in);
System.out.print("Enter two no a and b is : ");
a=sc.nextInt();
b=sc.nextInt();
System.out.println("Result of logical AND = "+(a&b));
System.out.println("Result of logical OR = "+(a|b));
System.out.println("Result of logical NOT = "+(~a));
System.out.println("Result of shift right = "+(a>>1));
System.out.println("Result of shift left = "+(a<<1));
System.out.println("a equal to b is : "+(a==b));
}
}
Output:
Enter two no a and b is : 10 5Result of logical AND = 0
Result of logical OR = 15
Result of logical NOT = -11
Result of shift right = 5
Result of shift left = 20
a equal to b is : false
Find the result of bitwise operators with user input at command line :
It means the value is assign on a and b variable at the command line.class math
{
public static void main(String args[])
{
int a,b;
a=Integer.parseInt(args[0]);
b=Integer.parseInt(args[1]);
System.out.println("Result of logical AND = "+(a&b));
System.out.println("Result of logical OR = "+(a|b));
System.out.println("Result of logical NOT = "+(~a));
System.out.println("Result of shift right = "+(a>>1));
System.out.println("Result of shift left = "+(a<<1));
System.out.println("a equal to b is : "+(a==b));
}
}
Output:
C:/>java math 45 5 Result of logical AND = 5Result of logical OR = 45
Result of logical NOT = -46
Result of shift right = 22
Result of shift left = 90
a equal to b is : false
0 Comments