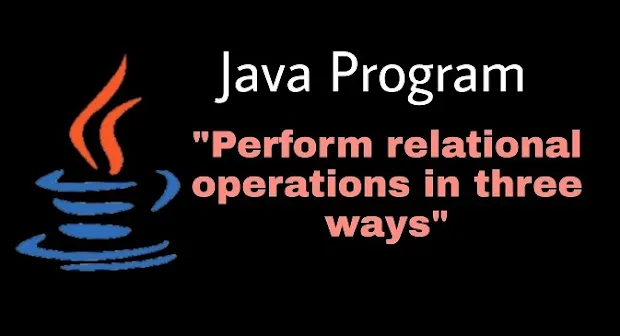
Three ways to perform relational operations:
- Without user input
- With user input at runtime
- With user input at command line
Perform relational operations without user input:
It means at the time of declaration of variable , value is assign on a and b variable. ‘a’ is an one variable and ‘b’ is another variable. ‘math’ is a class name.class math
{
public static void main(String args[])
{
int a=15, b=12;
System.out.println("a greater than b is :"+(a>b));
System.out.println("a less than b is :"+(a<b));
system.out.println("a greater than equal to b is :"+(a>=b));
System.out.println("a less than equal to b is :"+(a<=b));
System.out.println("a not equal to b is :"+(a!=b));
System.out.println("a equal to b is :"+(a==b));
}
}
Output:
a greater than b is : truea less than b is : false
a greater than equal to b is : true
a less than equal to b is : false
a not equal to b is : true
a equal to b is : false
Perform relational operations with user input at run time:
It means at the execution time the value is assign on a and b variable. This program use scanner class then import util package.import java.util.*;
class math
{
public static void main(String args[])
{
int a,b;
Scanner sc= new Scanner(System.in);
System.out.print("Enter two no a and b is : ");
a=sc.nextInt();
b=sc.nextInt();
System.out.println("a greater than b is :"+(a>b));
System.out.println("a less than b is :"+(a<b));
system.out.println("a greater than equal to b is :"+(a>=b));
System.out.println("a less than equal to b is :"+(a<=b));
System.out.println("a not equal to b is :"+(a!=b));
System.out.println("a equal to b is :"+(a==b));
}
}
Output:
Enter two no a and b is : 50 40a greater than b is : true
a less than b is : false
a greater than equal to b is : true
a less than equal to b is : false
a not equal to b is : true
a equal to b is : false
Perform relational operations with user input at command line :
It means the value is assign on a and b variable at the command line.class math
{
public static void main(String args[])
{
int a,b;
a=Integer.parseInt(args[0]);
b=Integer.parseInt(args[1]);
System.out.println("a greater than b is :"+(a>b));
System.out.println("a less than b is :"+(a<b));
system.out.println("a greater than equal to b is :"+(a>=b));
System.out.println("a less than equal to b is :"+(a<=b));
System.out.println("a not equal to b is :"+(a!=b));
System.out.println("a equal to b is :"+(a==b));
}
}
Output:
C:\>java math 75 90a greater than b is : false
a less than b is : true
a greater than equal to b is : false
a less than equal to b is : true
a not equal to b is : true
a equal to b is : false
0 Comments